<style>
.reveal section img { background:none; border:none; box-shadow:none; }
</style>
# GBA Workshop
----
## Hardware
- 32-bit ARMv4T CPU
- 240x160 pixel LCD
- 2D Video with bitmap and sprite modes
- 6 Audio Channels
- controlled via memory mapped I/O registers
- Buttons, Link port, ...
----
## Memory mapped I/O ?
Some special memory adress, that does not just store data, but controls peripherals when you read or write to it.
----
### GBA's memory MAP
```
General Internal Memory
00000000-00003FFF BIOS ROM (16 KBytes)
02000000-0203FFFF RAM (256 KBytes)
03000000-03007FFF Some faster RAM (32 KBytes)
>>> 04000000-040003FE I/O Registers <<<
Internal Display Memory
05000000-050003FF Color Palette (1 Kbyte)
06000000-06017FFF Video Memory (96 KBytes)
07000000-070003FF Sprite Attributes (1 Kbyte)
```
----
### GBA's I/O Registers
```
LCD I/O Registers
4000000h 2 R/W DISPCNT LCD Control
4000002h 2 R/W - Undocumented
4000004h 2 R/W DISPSTAT General LCD Status (STAT,LYC)
4000006h 2 R VCOUNT Vertical Counter (LY)
4000008h 2 R/W BG0CNT BG0 Control
400000Ah 2 R/W BG1CNT BG1 Control
400000Ch 2 R/W BG2CNT BG2 Control
400000Eh 2 R/W BG3CNT BG3 Control
...
```
----
### LCD Control
```
4000000h - DISPCNT - LCD Control (Read/Write)
Bit Expl.
0-2 BG Mode (0-5=Video Mode 0-5, 6-7=Prohibited)
3 Reserved / CGB Mode (0=GBA, 1=CGB; can be set only by BIOS opcodes)
4 Display Frame Select (0-1=Frame 0-1) (for BG Modes 4,5 only)
5 H-Blank Interval Free (1=Allow access to OAM during H-Blank)
6 OBJ Character VRAM Mapping (0=Two dimensional, 1=One dimensional)
7 Forced Blank (1=Allow FAST access to VRAM,Palette,OAM)
8 Screen Display BG0 (0=Off, 1=On)
9 Screen Display BG1 (0=Off, 1=On)
10 Screen Display BG2 (0=Off, 1=On)
11 Screen Display BG3 (0=Off, 1=On)
12 Screen Display OBJ (0=Off, 1=On)
```
---
## Example
### Getting pixels on the LCD
----
#### GBA Video
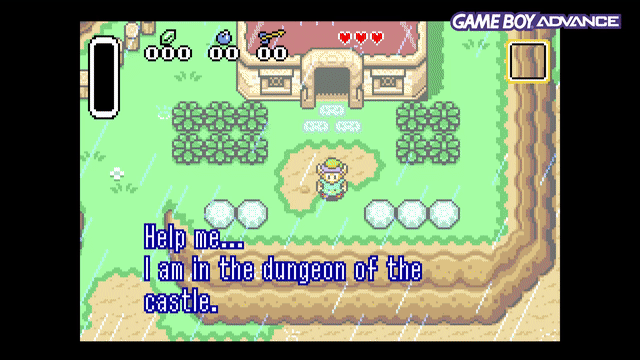
source > https://www.youtube.com/watch?v=mpNWEbZdXNw
----
#### The hard way
```
int main()
{
*(unsigned int*)0x04000000 = 0x0403;
((unsigned short*)0x06000000)[120+80*240] = 0x001F;
((unsigned short*)0x06000000)[136+80*240] = 0x03E0;
((unsigned short*)0x06000000)[120+96*240] = 0x7C00;
while(1);
return 0;
}
```
----
#### Compile and run >>
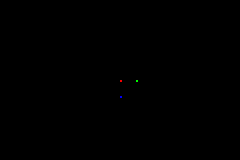
----
#### But why?
```
0x04000000 = 0x0403 ????
```
Reminder:
```
4000000h 2 R/W DISPCNT LCD Control
```
----
Configures LCD to use Mode 3
```
Bit Expl.
0-2 BG Mode (0-5=Video Mode 0-5, 6-7=Prohibited)
...
```
and to enable Background 2
```
Bit Expl.
8 Screen Display BG0 (0=Off, 1=On)
9 Screen Display BG1 (0=Off, 1=On)
10 Screen Display BG2 (0=Off, 1=On)
11 Screen Display BG3 (0=Off, 1=On)
```
0000 0100 0000 0011 == 0x0403
----
#### The C way / tonclib
```
#include "toolbox.h"
int main()
{
REG_DISPCNT= DCNT_MODE3 | DCNT_BG2;
m3_plot( 120, 80, RGB15(31, 0, 0) ); // or CLR_RED
m3_plot( 136, 80, RGB15( 0,31, 0) ); // or CLR_LIME
m3_plot( 120, 96, RGB15( 0, 0,31) ); // or CLR_BLUE
while(1);
return 0;
}
```
----
#### The C++ way / bugba
```
#include "gba_mem_sym.hpp"
int main()
{
using namespace gba::dtypes;
using namespace gba::hw;
reg_disp.lcd_conf = {
.mode = lcd_mode_t::mode3,
.bg2 = true,
};
mem_vram.mode3.pix[72][112] = {.red = 31};
mem_vram.mode3.pix[72][128] = {.green = 31};
mem_vram.mode3.pix[88][112] = {.blue = 31};
loop();
}
```
---
## Howto
----
### Install
- SDK https://devkitpro.org/wiki/Getting_Started
- (dkp-)pacman -Syu gba-dev
- Intro https://www.coranac.com/tonc/
- Docs https://problemkaputt.de/gbatek.htm
- flash https://github.com/lesserkuma/FlashGBX
- bugba https://github.com/deheisenbug/bugba
- edit Makefile.conf. uncomment #USE_DKP=1
- butano https://github.com/GValiente/butano
- rust https://github.com/rust-console/gba
- slides https://s.42l.fr/gbaworkshop
----
## Todo
- put all examples in one repo
{"title":"GBA Workshop","tags":"presentation","slideOptions":{"theme":"solarized","transition":"fade"}}